题目
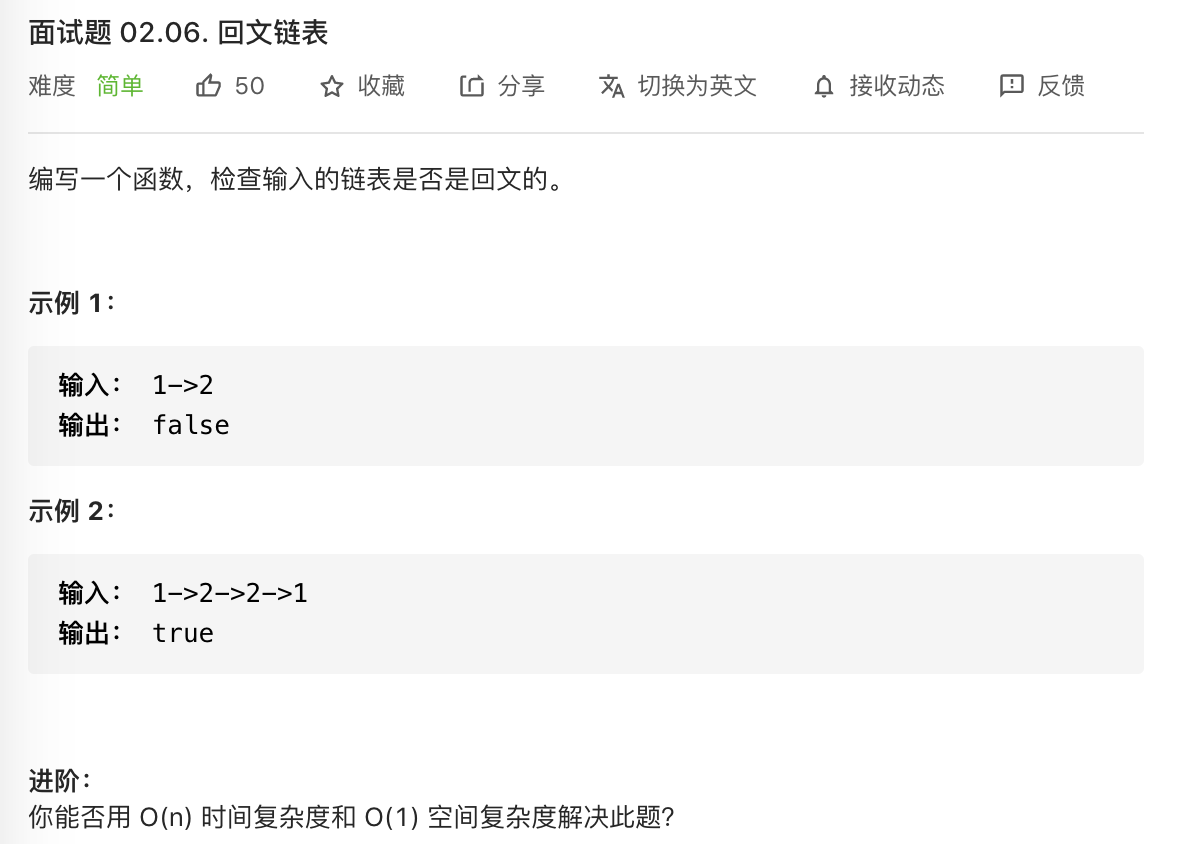
这个题用链表方式可以实现,但效率很低
因为是回文链表,使用快慢指针,每次循环让fast走两步,slow走一步
反转后半段链表,反转起始节点为当前slow节点
题目解答
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43
| public class Solution { public boolean isPalindrome(ListNode head) { ListNode slow = head; ListNode fast = head;
while (slow != null && fast != null && fast.next != null) { slow = slow.next; fast = fast.next.next; }
ListNode newHead = reverseList(slow);
while (newHead != null && head != null) { if (newHead.val != head.val) { return false; }
newHead = newHead.next; head = head.next; } return true; }
public ListNode reverseList(ListNode head) { return reverseList(head, null); }
public ListNode reverseList(ListNode head, ListNode newHead) {
if (head == null) { return newHead; }
ListNode tmp = head.next;
head.next = newHead; newHead = head;
return reverseList(tmp, newHead); } }
|